- Published on
Sending and Receiving Messages with Node.js + RabbitMQ
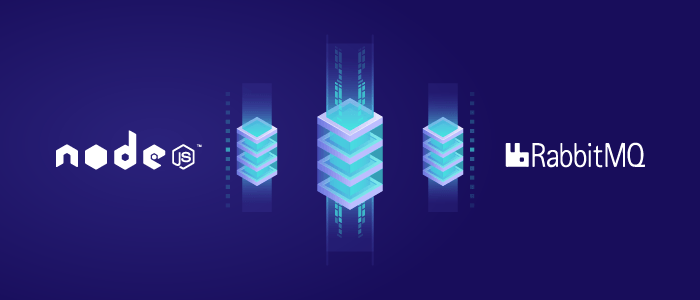
Continuing from my previous post Installing and Configuring RabbitMQ Message Broker, I will explain how to implement it with Node.js using the Async/Await method. In Node.js, there are two concepts: Asynchronous and Synchronous. To make it easier to understand, let me use an analogy. Asynchronous is like a waiter in a restaurant with two customers, Customer A and Customer B. The waiter first serves Customer A, then hands the order to the kitchen. While waiting for Customer A’s order to be cooked, the waiter can continue serving Customer B and hand their order to the kitchen as well. In contrast, with Synchronous, the waiter must wait until Customer A’s order is fully cooked and served before they can continue serving Customer B. Personally, I prefer the asynchronous concept because we don’t have to wait for a command to complete.
Alright, that’s enough small talk—let’s move on to coding!
Step 1: Install the amqp Library
We need a library to send messages to RabbitMQ in Node.js using amqplib.
Install using npm
$ npm i amqplib
Step 2: Create a Producer
Create a new file named send.js
. Here, I will use JSON as the message payload to make it more flexible for a single message transmission.
Step 3: Create a Consumer
After finishing the message sender, create a receiver for the messages.
Create a new file named receive.js
.
Step 4: Execute
After creating the sender and receiver scripts, run the message receiver script first.
$ node receive.js
Listening for messages...
Then run the message sender script
$ node send.js "Hai"
[x] Sent: Hai
A new message will appear in receive.js that you ran earlier
Listening for messages...
nama-antrian:'{"message":"Hai"}'
Additional
To view the list of queues, you can use the following command in a Linux terminal:
$ sudo rabbitmqctl list_queues
Or in the Windows command prompt:
> rabbitmqctl.bat list_queues
Or you can open the URL below if using the web GUI.
http://{hostname}:15672/#/queues
That’s it, see ya~